Решил сделать сортировку инвентаря в Interlude подсмотрел в других исходниках, делают по весу я сделал также но постарался написать свой код как я вижу.
Но вот вопрос как всё таки правильно сортировать предметы? я думаю по весу это не правильный подход, так как есть еще заточка, грейд и тп.
Как вы сортируете свой инвентарь?
У меня получилось та(
Свой срипт: aaIT_SortInventory.uc
Но вот вопрос как всё таки правильно сортировать предметы? я думаю по весу это не правильный подход, так как есть еще заточка, грейд и тп.
Как вы сортируете свой инвентарь?
У меня получилось та(
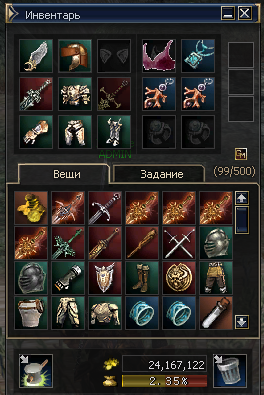
Свой срипт: aaIT_SortInventory.uc
C++:
/******************************************
Дата 28.07.2024 13:54
Разработчик: BITHACK
Copyright (c) Ваша компания
Описание скрипта: Сортировка инвентаря по типам предметов и весу.
Сначала сортировка по типам:
- Ассеты
- Оружие
- Броня
- Аксессуары
- Прочие предметы (в том числе экипировка, зелья, стрелы, рецепты, материалы)
Затем сортировка каждого типа по весу.
*******************************************/
class aaIT_SortInventory extends UICommonAPI;
var int nextSlot;
var ItemWindowHandle ItemWnd;
//! Сортировка инвентаря
function SortItem(ItemWindowHandle m_invenItem)
{
local int i;
local int invenLimit;
local ItemInfo item;
local EItemType eItemType;
// Массивы для хранения элементов каждого типа
local Array<ItemInfo> AssetList;
local Array<ItemInfo> WeaponList;
local Array<ItemInfo> ArmorList;
local Array<ItemInfo> AccesaryList;
local Array<ItemInfo> EtcItemList;
// Массивы для хранения элементов "прочих предметов"
local Array<ItemInfo> BlessEnchantAmList;
local Array<ItemInfo> BlessEnchantWpList;
local Array<ItemInfo> EnchantAmList;
local Array<ItemInfo> EnchantWpList;
local Array<ItemInfo> PotionList;
local Array<ItemInfo> ElixirList;
local Array<ItemInfo> ArrowList;
local Array<ItemInfo> RecipeList;
local Array<ItemInfo> MaterialList;
ItemWnd = m_invenItem;
invenLimit = ItemWnd.GetItemNum();
// Сортировка по типу
for (i = 0; i < invenLimit; ++i)
{
ItemWnd.GetItem(i, item);
eItemType = EItemType(item.ItemType);
switch (eItemType)
{
case ITEM_ASSET:
AssetList.Length = AssetList.Length+1;
AssetList[AssetList.Length-1] = item;
break;
case ITEM_WEAPON:
WeaponList.Length = WeaponList.Length+1;
WeaponList[WeaponList.Length-1] = item;
break;
case ITEM_ARMOR:
ArmorList.Length = ArmorList.Length+1;
ArmorList[ArmorList.Length-1] = item;
break;
case ITEM_ACCESSARY:
AccesaryList.Length = AccesaryList.Length+1;
AccesaryList[AccesaryList.Length-1] = item;
break;
case ITEM_ETCITEM:
switch (EEtcItemType(item.ItemSubType))
{
case ITEME_ENCHT_AM:
EnchantAmList.Length = EnchantAmList.Length+1;
EnchantAmList[EnchantAmList.Length-1] = item;
break;
case ITEME_BLESS_ENCHT_AM:
BlessEnchantAmList.Length = BlessEnchantAmList.Length+1;
BlessEnchantAmList[BlessEnchantAmList.Length-1] = item;
break;
case ITEME_ENCHT_WP:
EnchantWpList.Length = EnchantWpList.Length+1;
EnchantWpList[EnchantWpList.Length-1] = item;
break;
case ITEME_BLESS_ENCHT_WP:
BlessEnchantWpList.Length = BlessEnchantWpList.Length+1;
BlessEnchantWpList[BlessEnchantWpList.Length-1] = item;
break;
case ITEME_POTION:
PotionList.Length = PotionList.Length+1;
PotionList[PotionList.Length-1] = item;
break;
case ITEME_ELIXIR:
ElixirList.Length = ElixirList.Length+1;
ElixirList[ElixirList.Length-1] = item;
break;
case ITEME_ARROW:
ArrowList.Length = ArrowList.Length+1;
ArrowList[ArrowList.Length-1] = item;
break;
case ITEME_RECIPE:
RecipeList.Length = RecipeList.Length+1;
RecipeList[RecipeList.Length-1] = item;
break;
case ITEME_MATERIAL:
MaterialList.Length = MaterialList.Length+1;
MaterialList[MaterialList.Length-1] = item;
break;
default:
EtcItemList.Length = EtcItemList.Length+1;
EtcItemList[EtcItemList.Length-1] = item;
break;
}
break;
default:
EtcItemList.Length = EtcItemList.Length+1;
EtcItemList[EtcItemList.Length-1] = item;
break;
}
}
// Сортировка списков по весу
SortListByWeight(AssetList);
SortListByWeight(WeaponList);
SortListByWeight(ArmorList);
SortListByWeight(AccesaryList);
SortListByWeight(EnchantAmList);
SortListByWeight(BlessEnchantAmList);
SortListByWeight(EnchantWpList);
SortListByWeight(BlessEnchantWpList);
SortListByWeight(PotionList);
SortListByWeight(ElixirList);
SortListByWeight(ArrowList);
SortListByWeight(RecipeList);
SortListByWeight(MaterialList);
SortListByWeight(EtcItemList);
// Добавление элементов в инвентарь
AddItems(AssetList);
AddItems(WeaponList);
AddItems(ArmorList);
AddItems(AccesaryList);
AddItems(EnchantAmList);
AddItems(BlessEnchantAmList);
AddItems(EnchantWpList);
AddItems(BlessEnchantWpList);
AddItems(PotionList);
AddItems(ElixirList);
AddItems(ArrowList);
AddItems(RecipeList);
AddItems(MaterialList);
AddItems(EtcItemList);
}
// Функция для сортировки списка по весу
function SortListByWeight(out array<ItemInfo> ItemList)
{
local int i, j;
local ItemInfo temp;
for (i = 0; i < ItemList.Length - 1; ++i)
{
for (j = i + 1; j < ItemList.Length; ++j)
{
if (ItemList[i].Weight > ItemList[j].Weight)
{
temp = ItemList[i];
ItemList[i] = ItemList[j];
ItemList[j] = temp;
}
}
}
}
// Функция добавление элементов в инвентарь
function AddItems(array<ItemInfo> ItemList)
{
local int i;
for (i = 0; i < ItemList.Length; i++)
{
ItemWnd.SetItem(nextSlot, ItemList[i]);
nextSlot++;
}
}
defaultproperties{}